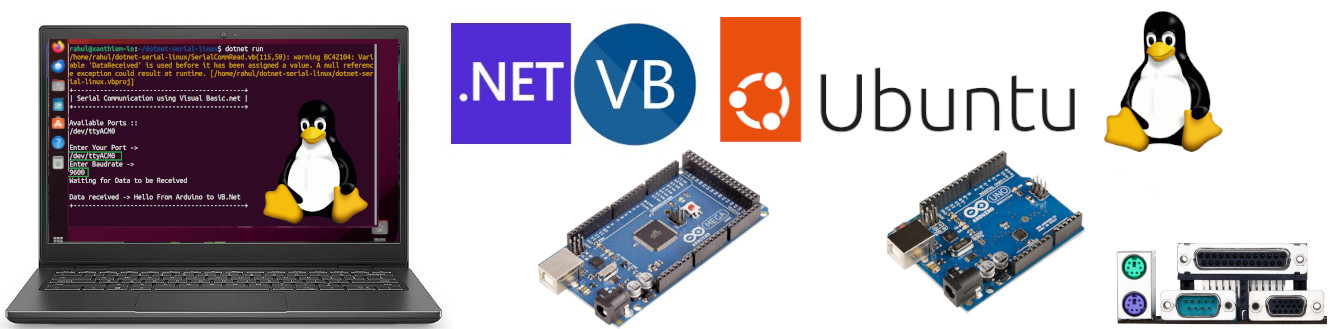
Following the introduction of the open-source .NET platform by the .NET Foundation, supported by Microsoft, it became possible to develop .NET applications on platforms other than Windows, including Linux and macOS, using languages such as C# and Visual Basic .NET.
Before this, developers primarily relied on the Mono Framework from Xamarin for developing .NET applications on non-Windows platforms. With Microsoft's release of its .NET platform, installing the framework on various platforms has been streamlined for users.
In this tutorial we will learn to program the serial port of a Linux system using Visualbasic.net and the .NET Platform Runtime. We will send and receive data from a microcontroller board like Arduino to a Linux PC using Virtual Serial Port Connection (VCP).
Contents
- VB.Net Source Codes
- Install .NET SDK CLI on Linux
- Creating VB.net Project on Linux using .NET SDK
- Installing System.IO.Ports namespace on Linux using Nuget
- Identifying Serial Port Name/Number on Linux
- Serial Port Permissions in Linux
- Opening a Serial Port on Linux using vb.net
Source Codes
Download Source codes for cross platform vb.net serial port programming as Zip file
Source codes for cross platform vb.net serial port programming can be found from our Github Repo
Install .NET SDK CLI on Linux
You can install the dotnet (.NET) SDK on Linux using the below command. Here I am using Ubuntu Linux. SDK will also install the dotnet runtime.
sudo apt-get install -y dotnet-sdk-8.0

Here dotnet-sdk-8.0 is the name of the SDK and may change in the future. Replace it with the correct version number of the latest available SDK.
The -y option in the apt-get install command stands for "yes". When you use -y, you're telling the system to automatically answer "yes" to any prompts or confirmation messages that may appear during the installation process. This is useful when you want to automate the installation of packages and don't want to manually confirm each installation step
You can check the installed dotnet SDK using the following command.
dotnet --list-sdks
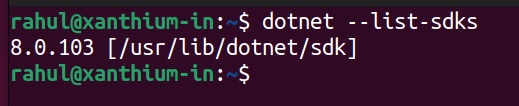
You can also view the installed dotnet runtimes using the below command.
dotnet --list-runtimes

Creating VB.net Project on Linux using .NET SDK
After the .NET SDK and .NET runtime have been installed on your Linux system.
You can create a simple VB.net project to develop software targeting the .NET (dotnet) platform on Linux systems like Ubuntu, Centos, Rocky Linux ,Debian, Fedora etc.
Here we will be creating a vb.net console project using the command line tools provided by the dotnet sdk. Currently WinForms Project and WPF project types are not supported on Linux systems by the .NET SDK due to the tight integration of these projects to the underlying Win32 API calls.
You can do that by issuing the following command
dotnet new console --language VB -o directory_to_store_project_files
here programming language ,we want use is Visual Basic.net so --language VB flag.
-o flag creates a directory to store the project files.
Here we are going to name the directory as dotnet-serial-linux
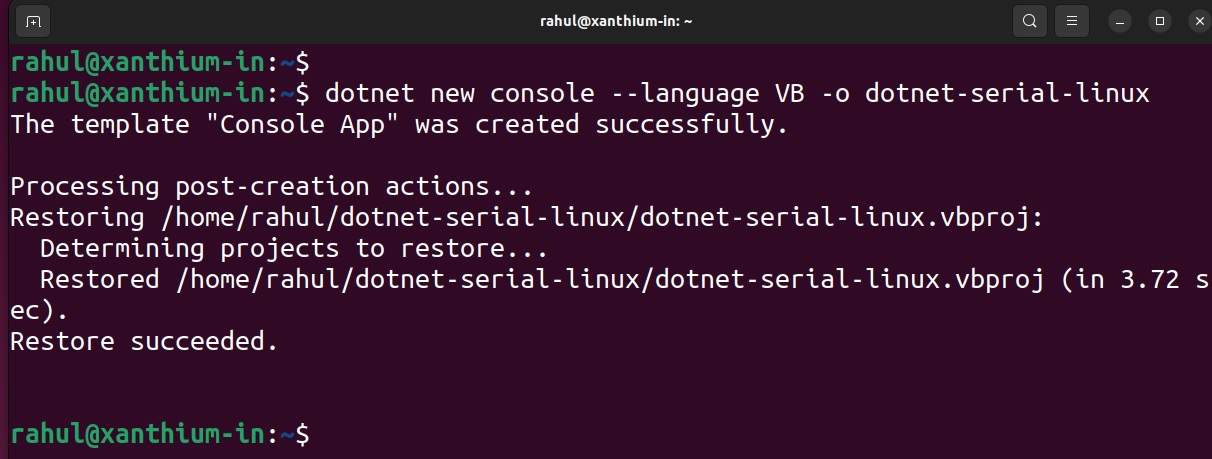
You can now change into the dotnet-serial-linux directory and type
dotnet run
to run the VisualBasic.net code. It will just print a Hello World! as shown below.

Installing System.IO.Ports namespace on Linux.
On .NET Platform, the System.IO.Ports namespace which contain the SerialPort class is not included by default.
You have manually install the System.IO.Ports namespace and the associated assemblies to your serial port programming project using the Nuget package manager.
You can go to nuget.org website and search for the System.IO.Ports package and then install it.
The install command can be copied directly from the website. You can also select different version of the package. Here we are using version 8.0.0
The command is
dotnet add package System.IO.Ports --version 8.0.0
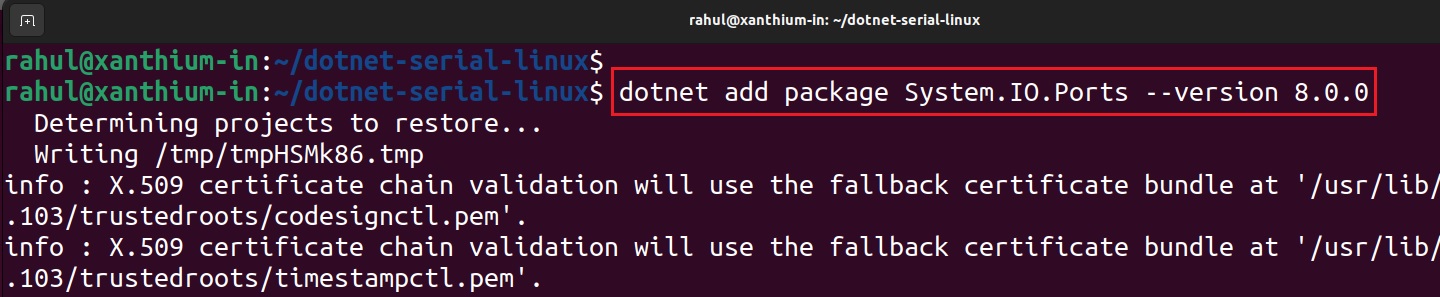
Now that the System.IO.Ports package is installed for your current project ,you can access the SerialPort class from your VB.net program.
Identifying Serial Ports on Linux
The naming conventions for serial ports vary between Linux and Windows. In Windows, ports are labeled as COM1, COM22, COM34, etc., following the COMxx system.
However, in Linux and similar operating systems, hardware ports are typically named ttyS1, ttyS2, etc., while virtual serial ports generated by USB to serial converter chips are labeled as ttyUSB0, ttyUSB1, or ttyACM0, ttyACM1, etc.
To connect/open the serial port , you have to give the full name like
- /dev/ttyS1
- /dev/ttyUSB0
- dev/ttyACM0
For example
my_linux_serial_port = New SerialPort()
my_linux_serial_port.PortName = "/dev/ttyUSB0"
Unlike Windows, Linux is case sensitive so make sure that you are using the correct case while entering the serial port name/number.
Now connect the USB device (here an Arduino UNO) to your Linux PC and issue the following commands.
sudo dmesg | tail
dmesg is a command used in Linux to display the message buffer of the kernel. Message buffer contains information about the various hardware components connected to the PC as well as the device drivers loaded to communicate with the hardware. The dmesg command allows users to view this buffer, providing information about hardware detection, driver initialization, and any errors or warnings encountered during the boot process or while the system is running.
The output of this is passed to the tail command which is used to display the last and most recent messages generated by the Kernel which corresponds to the connection of Arduino UNO.
The output will look something like this.
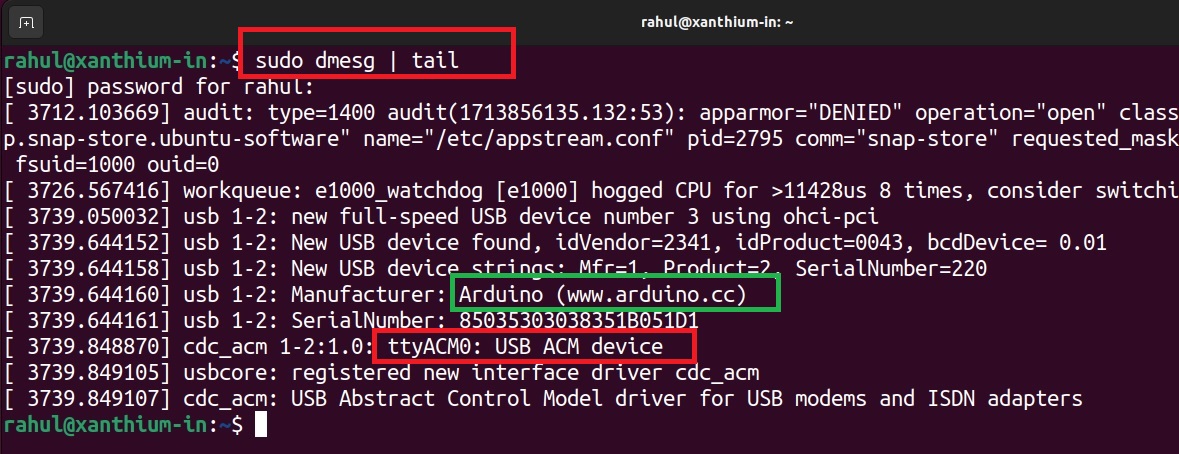
So in this case the name of our serial port is ttyACM0 or /dev/ttyACM0.
You can also get the connected serial ports on a Linux System by running the below VisualBasic.net code that targets the .NET platform.
'Source code name-> FindAvailablePorts.vb
Imports System.IO.Ports 'For accessing the SerialPort Class and Methods
Module AvailablePorts
Sub Main()
Dim AvailablePorts() As String = SerialPort.GetPortNames() 'List available ports on a System
Dim Port As String
Console.WriteLine("Available Ports ::")
For Each Port In AvailablePorts
Console.WriteLine(Port)
Next Port
End Sub
End Module
Here is the output of the code running on Ubuntu Linux system.
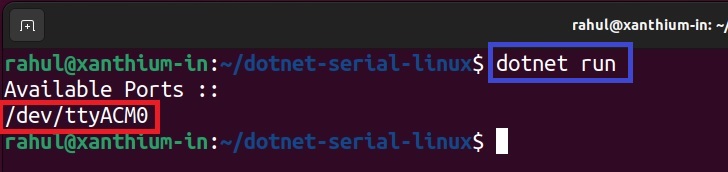
Serial Port Permissions in Linux
Linux do not allow a user to access the serial port (tty) unless they are part of a specific group like tty,dialout or uucp.
You can use the groups command to check whether your account is part of the above mentioned groups.
Please issue the command
groups username

From the above image ,the user "rahul" is not part of groups tty,dialout and uucp.This means that the user rahul will not be allowed to open the serial port.
If you try to open a serial port without setting the correct permissions, you will get a Access to Port "/dev/ttyACM0"is denied error.
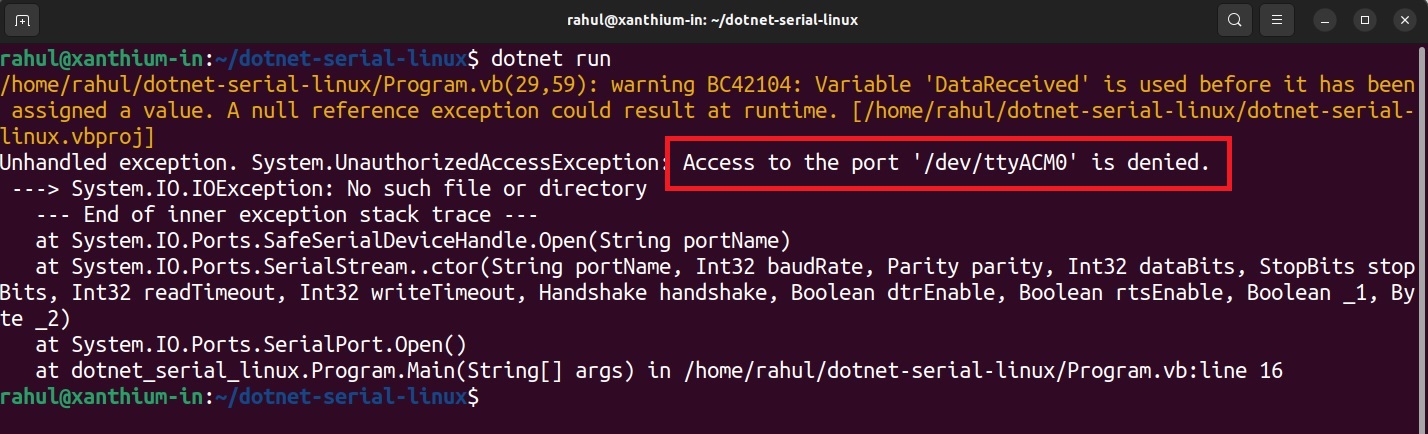
To solve the above error you will have to add the user to the relevant groups using usermod command as shown below
sudo usermod -a -G <group_name> <username>
So in our case
sudo usermod -a -G tty rahul
sudo usermod -a -G dialout rahul
sudo usermod -a -G uucp rahul
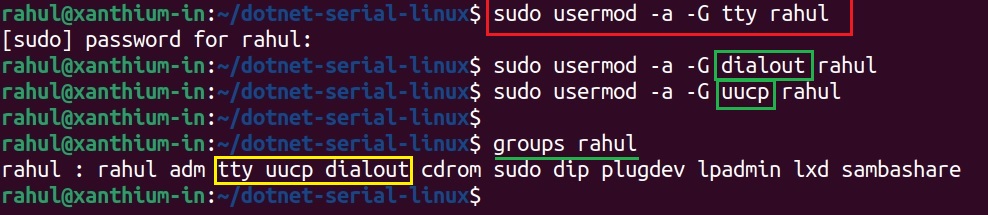
then log off from your Linux system and log back in for the changes to take effect.
Opening a Serial Port on Linux
Opening a serial port, setting parameters of Serial port , reading and writing data to the serial port are similar to how it is done on a windows platform as we are using the same API's. Here I wont delve deep into the intricacies of opening the serial port and will just run the code from our Github repo.
- You can read the detailed article on programming the various parameters of Serial Port using VB.net and communicating with an Arduino here.
Please note that opening the serial port on Linux using Visualbasic.net may reset the connected Arduino Board. Make sure that Arduino code is written to accommodate this condition.
Hardware connections are shown below.
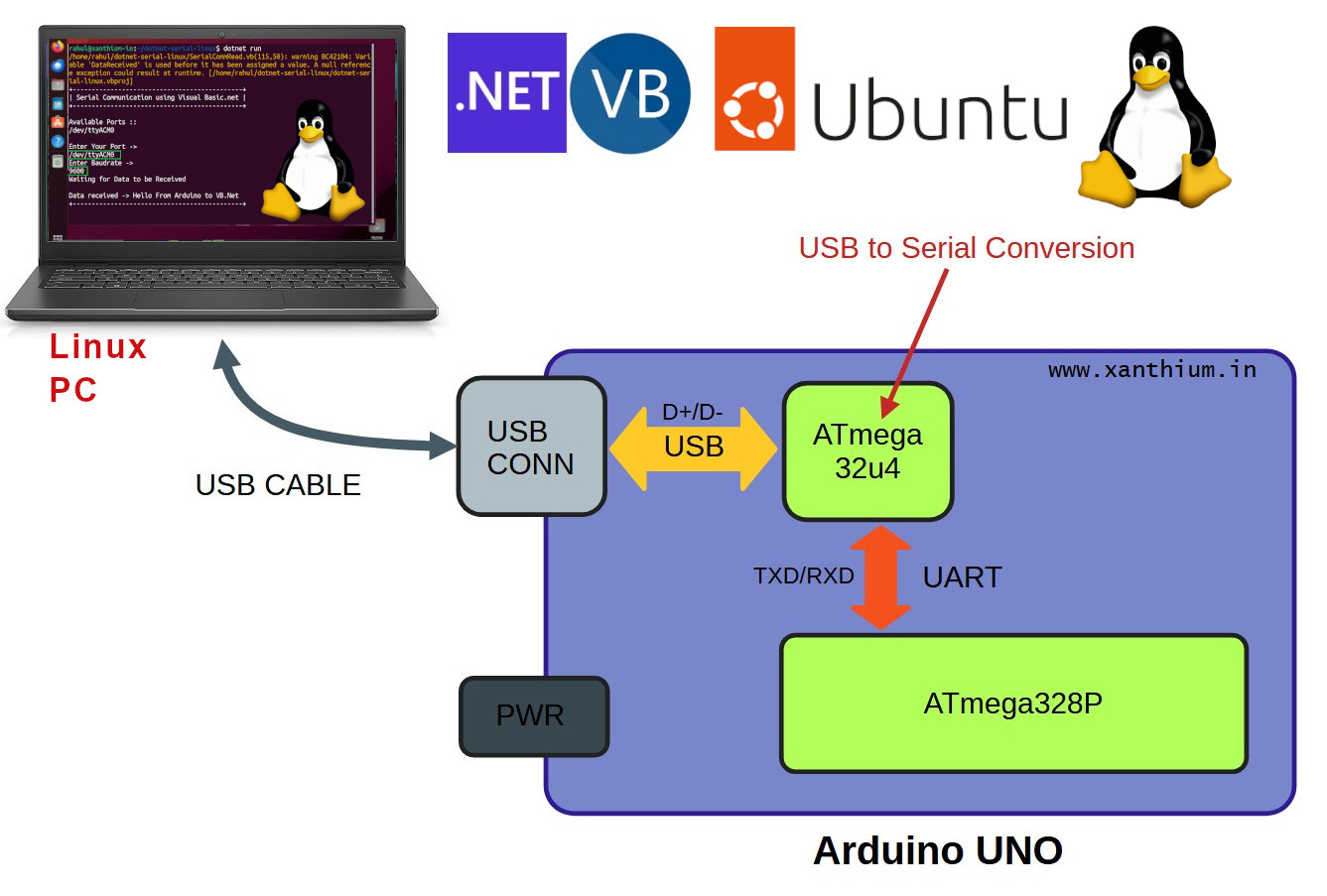
You can identify the serial port number of Arduino by using the dmesg command as shown in the tutorial (above).
Now you can run the SerialCommRead.vb file which would receive a string from Arduino and display it on the Linux Console. Copy the file inside the working directory.Remove any other vb files from the directory. Now use dotnet run to execute the file.
When you are giving the name of the serial port make sure you use the full name like /dev/ttyACM0.
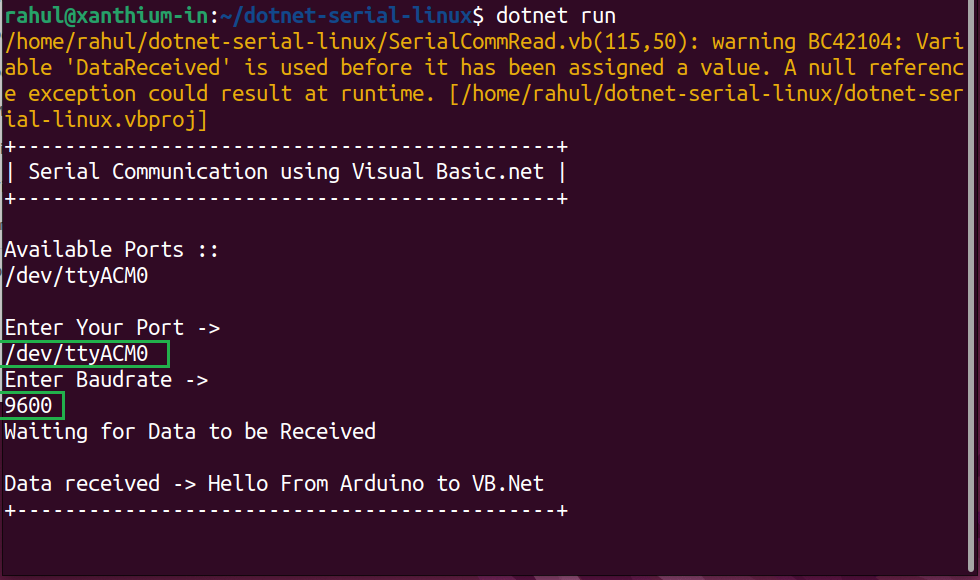
- Log in to post comments