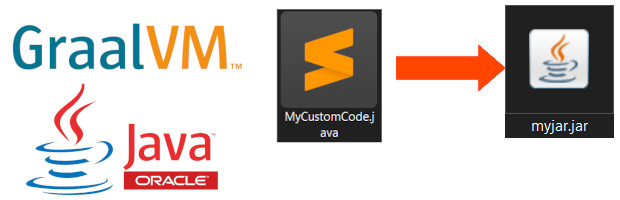
In this tutorial,
we will learn to create executable JAR files using GraalVM Java VM on Windows platform.
GraalVM is a Java VM and JDK based on HotSpot/OpenJDK, implemented in Java. It supports additional programming languages like Java,Python, Ruby, Javascript R and execution modes, like ahead-of-time compilation of Java applications for fast startup and low memory footprint.
All these examples will work with Oracle Java Development Kit too.
Contents
- Downloading GraalVM JVM
- Installing and running GraalVM JVM
- Source Codes
- Compiling and Running Java Source file
- Using packages in Java Programs
- Using the Java JAR file
- Creating a JAR file using GraalVM / Oracle JDK
- Creating an executable JAR file using GraalVM /Oracle JDK
- Simple class Example
- Example with hierarchical package classes
- Using JAR file Library in your Java Source code
- Compiling and running JAR files on Linux
Downloading GraalVM JVM
Installing and running GraalVM JVM on Windows
You can download GraalVM JVM from the link above in the form of ZIP file. Unzip the archive on location of your choice.
We won't be adding the java and javac compiler of GraalVM to the system path and will use the full path while compiling and running the code. So compiling and running the code will look like this.
H:\graalvm\bin\javac MyJavaSource.java
H:\graalvm\bin\java MyJavaSource
Reason i am doing this is that ,i already have Oracle Java SDK installed and want to make sure that i am using GraalVM SDK (java and javac) while compiling and running the code.
H:\graalvm\bin\
is the location of the downloaded the GraalVM SDK ,It may be different on your System.
Source Codes
For this tutorial we will be creating three java files.
- JMainEntry.java - contains the main class
- DBaccess.java - public dummy class DBaccess
- Serial.java - public dummy class Serial
- Download Java Source Zip Files
Compiling and Running java Source files using GraalVM / Oracle JDK
Tutorial is also available as a Video on YouTube.
You can compile and run these java files
H:\graalvm\bin\javac JMainEntry.java
H:\graalvm\bin\java JMainEntry
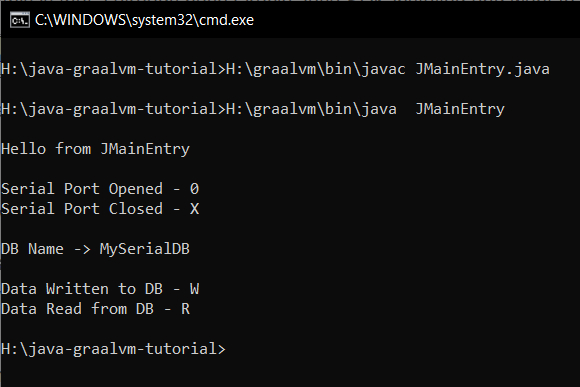
In the above example all the source files and class files created are placed in the same directory (java-graalvm-tutorial).
In the next section ,we will organize our java class files and source files in separate directories and compile them.
We will create two directories
- java-classes - to store our compiled classes
- java-sources - Source files
inside the java-graalvm-tutorial directory. Now our directory structure looks like this.
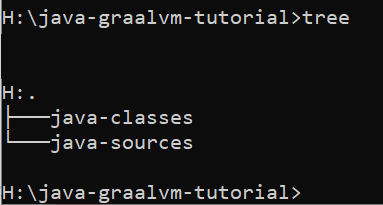
To compile the java Source files in the directory(java-sources), we can use the javac command as shown below,
Here we are using the * wild card character to compile all the .java files inside the folder.
H:\graalvm\bin\javac ./java-sources/*.java
issue with the above command is that resulting class files will be created inside the java-sources directory.
we can use the -d flag of the javac compiler to specify where to store our compiled class files.
H:\graalvm\bin\javac ./java-sources/*.java -d ./java-classes

After executing you will have the class files inside the java-sources directory as shown below.
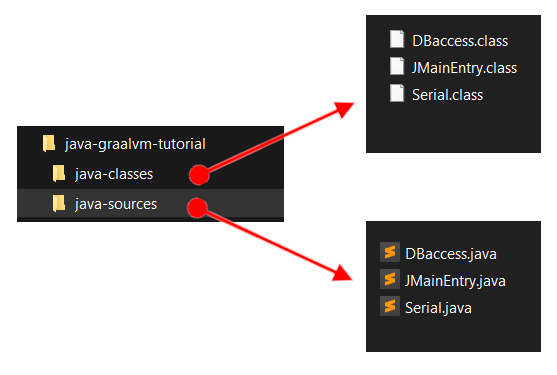
You can run the compiled class file using the below command.
H:\graalvm\bin\java -cp .\java-classes JMainEntry
Here we are using the classpath -cp flag to tell java JVM where to find our compiled class file JMainEntry.
Classpath -cp is followed immediately by the location of the class containing main().

Using packages in Java Programs
In this section we will learn how to build packages using command line tool from JDK (GraalVM/Oracle JDK).
If you prefer tutorial in Video format ,you can check the below YouTube video
A Java package is a way to organize classes, interfaces in Java.
We add the keyword package followed by package name to top of all three of your source files as shown below.
//JMainEntry.java
package com.mypackage; //package name
public class JMainEntry
{
public static void main (String[] Args)
{
//code
}
}
//Serial.java
package com.mypackage; //package name
public class Serial
{
//code
}
DBaccess.java
package com.mypackage; //package name
public class DBaccess
{
//code
}
Compiling the above files using the javac will create a directory structure like com/mypackage/ "compiled classes"
H:\graalvm\bin\javac -d . .\java-sources\*.java
//to put the compiled classes in Folder java-classes
H:\graalvm\bin\javac -d .\java-classes .\java-sources\*.java
You can RUN the code using
H:\graalvm\bin\java com.mypackage.JMainEntry
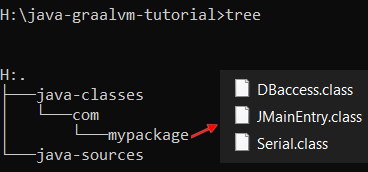
H:\graalvm\bin\java -cp .\java-classes com.mypackage.JMainEntry
Creating a JAR file using GraalVM / Oracle JDK
You can create a JAR file using the jar utility of the GraalVM JDK.
The command for creating the JAR file is shown below.(jar cvf)
jar cvf your-jar-file-name.jar input-files
- input-files can be java class files, images icons or other files you want to include with your program
- your-jar-file-name.jar The name you want to give to your jar file.
To view the contents of your JAR file you can use the tf option as shown below.
jar tf your-jar-file-name.jar
More details about jar file can be found at the oracle's website
Now i have copied all the three class files to a directory and will use the jar utility to pack them into a jar file called my-jar-1.0.jar
H:\graalvm\bin\jar cvf my-jar-1.0.jar *
Here i am using the * wild card character to tell the jar utility to pack all the files in the directory .
After creating, you can view the contents of your jar file using the tf option as shown below.
H:\graalvm\bin\jar tf my-jar-1.0.jar
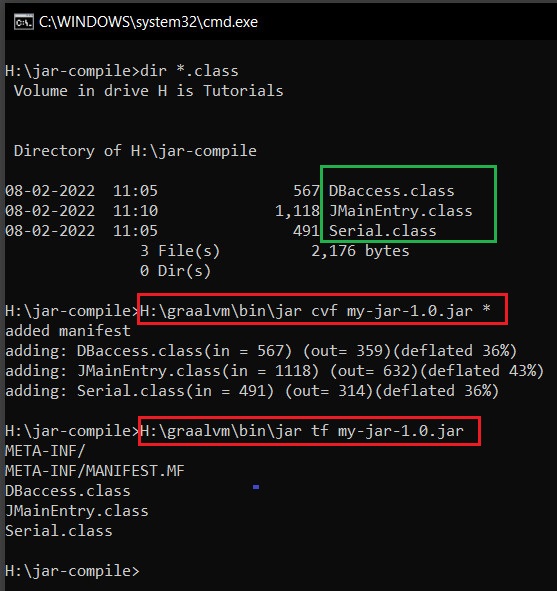
Please note that the JAR file you created will not be able to run since we have not set the entry point of our program in the MANIFEST file.
If you try to run the jar file ,you will get an error "no main manifest attribute, in my-jar-1.0.jar".
Creating an executable JAR file using GraalVM/Oracle JDK
To create an executable JAR file you will have to tell the entry point of the program or which class contains the main() function.
This can be done by the following option.
jar cvfe name-of-your-jar.jar ClassHavingMainFunc Location\your\myclass.class
For example, consider a simple code like
HelloWorld.java
public class HelloWorld
{
public static void main (String[] Args)
{
System.out.println("HelloWorld");
}
}
Place it inside a directory and compile the source code to .class file .
Now create the executable JAR file using the below command
jar cvfe HelloJar.jar HelloWorld HelloWorld.class
You can then run the resulting jar file by
java -jar HelloJar.jar
Now if you unzip the JAR file and view its MANIFEST.MF file
Manifest-Version: 1.0
Created-By: 17.0.2 (GraalVM Community)
Main-Class: HelloWorld
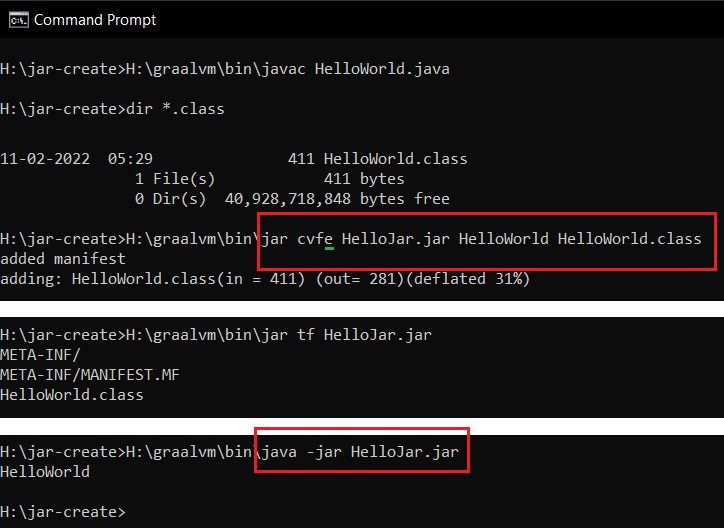
In the next example ,
We will learn how to create a multi file class JAR package containing hierarchical package name spaces.
All our source codes belong to the package com.mypackage
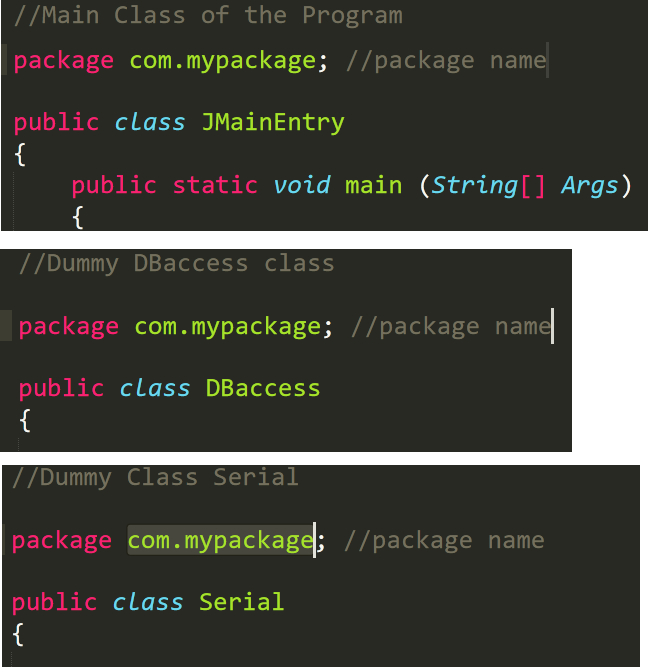
Now we put all these file in a directory and use the javac compiler to compile to .class files.
javac -d . *.java
this would create a directory structure com\mypackage\all your class files
You can run the class file by
java com.mypackage.JMainEntry
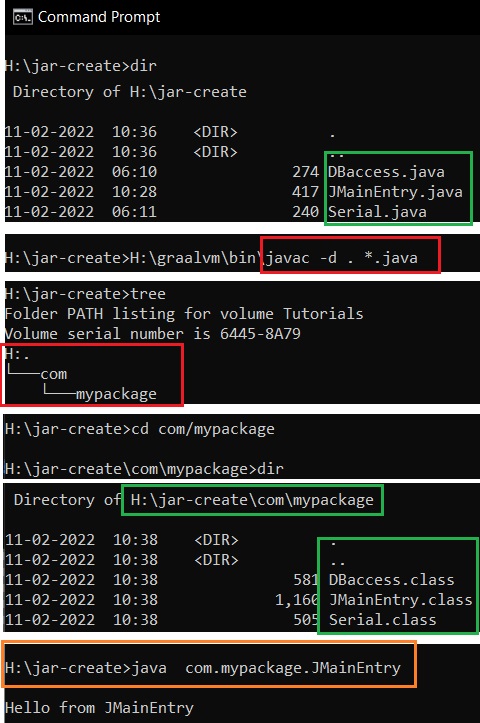
Now let's create an executable jar file for this. In the directory execute the below command.
jar cvfe MyPackageJar.jar com.mypackage.JMainEntry com\mypackage\*.class
After this you can execute the jar file using
java -jar MyPackageJar.jar
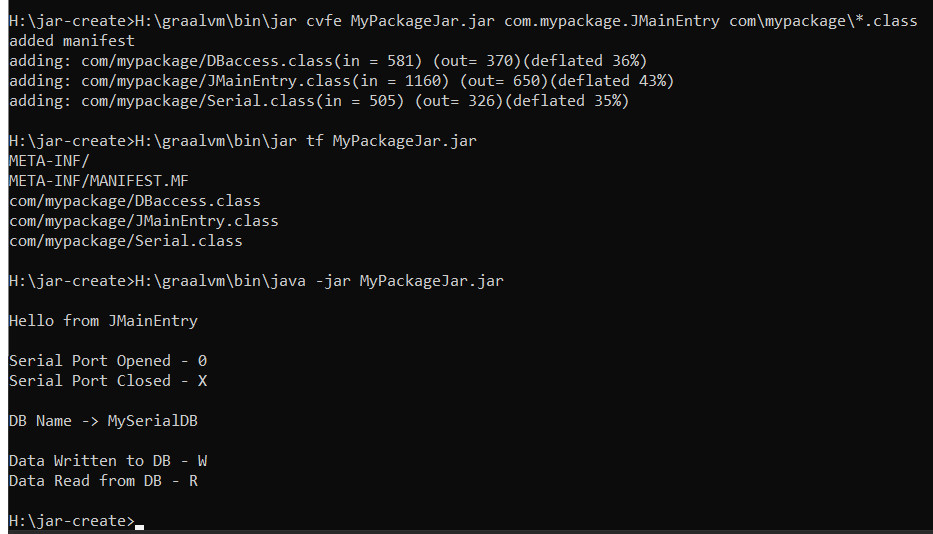
Using Java classes from a JAR library
Some times classes are distributed in the form of JAR files. In this tutorial we will learn to include classes inside the JAR file in our custom java program.
First we will create a custom JAR library using our Serial.java and DBaccess.java files.
Make sure that all the methods() in Serial.java and DBaccess.java are public.
We will compile them into class files and encapsulate them inside a JAR library called MyJARLib.jar.
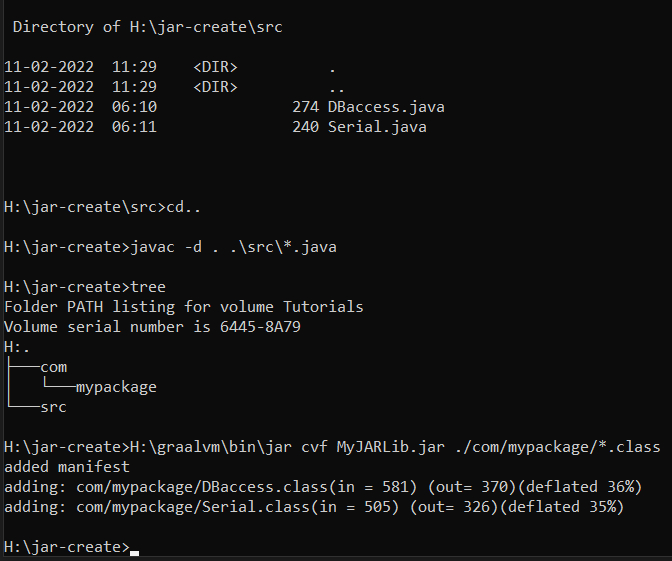
Please note that we are using only jar cvf flags as we do not want to execute the jar file.
Once the jar Library is created ,you can compile your own custom code which uses the classes inside the JAR file MyJARLib.jar.
Now create a .java file called MyCustomCode.java that will use the classes inside the jar file.
// MyCustomCode.java
//Main Class of the Program
//MyJARLib.jar
import com.mypackage.Serial; // Classes inside JAR
import com.mypackage.DBaccess; // Classes inside JAR
public class MyCustomCode
{
public static void main (String[] Args)
{
Serial S = new Serial(); //Class inside JAR
S.open();
}
}
You can compile the code as shown below,

//Compiling Code with JAR library
javac -cp .;MyJARLib.jar MyCustomCode.java
Running the Java Code with JAR library
java -cp .;MyJARLib.jar MyCustomCode
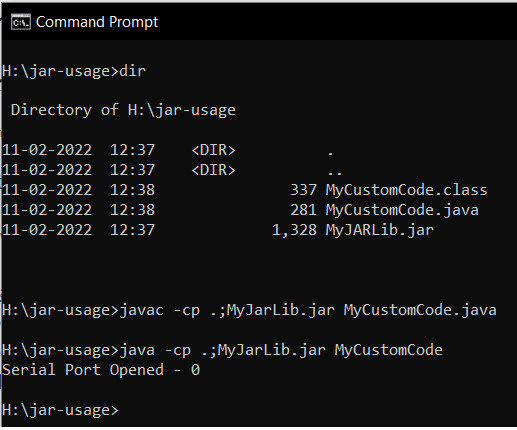
Compiling and running Java JAR files on Linux system
Please note that on Linux systems ,
you should replace semicolon ; with colon :
javac -cp .:MyJARLib.jar MyCustomCode.java
java -cp .:MyJARLib.jar MyCustomCode
- Log in to post comments