In this tutorial we will learn how to convert a tkinter (ttkbootstrap) python script (.py) to a Windows executable (.exe) using Pyinstaller
Contents
- What is PyInstaller
- Limitations of PyInstaller
- Installing Pyinstaller
- Convert Python (.py) file to Windows (.exe) file
- Creating a Single Windows Executable from Python Script
- Convert a tkinter python script to .exe file
- Convert ttkbootstrap Python script to a Windows exe
- Add Custom icon to Pyinstaller Generated Windows Executables
What is PyInstaller
PyInstaller is a popular opensource Python packaging tool used to convert Python applications into standalone executables. It allows you to distribute your Python applications as single, self-contained executables that can run on machines without needing a Python interpreter or any dependencies installed.
Key features of PyInstaller:
Cross-platform: PyInstaller supports packaging applications for multiple platforms, including Windows, macOS, and Linux. You can build executables for different platforms from a single machine.
Dependency management: PyInstaller analyzes your Python code to automatically detect and bundle any dependencies, including third-party libraries and resources such as images or data files. This helps ensure that your application runs smoothly on other machines without the need for manual dependency installation.
Freezing: PyInstaller "freezes" your application into a single executable file, along with the Python interpreter and any necessary support files. This process encapsulates your entire application into a single package, making it easy to distribute and deploy.
Optimization: PyInstaller offers options for optimizing the size and performance of the generated executables, such as compressing files, removing debugging information, or optimizing bytecode.
Opensource: PyInstaller is open-source software. It is released under the GNU General Public License (GPL), which means that its source code is freely available for anyone to view, modify, and distribute
Overall, PyInstaller simplifies the distribution of Python applications by providing a straightforward way to package them into standalone executables, eliminating the need for end-users to install Python or any dependencies manually.
Limitations
PyInstaller enables the creation of executables for Windows, Linux, and macOS individually, but it lacks cross-compilation capabilities. This means you cannot generate an executable targeting one operating system from another.
For Example, For generating a Windows executable you need a Windows machine, You cannot create a Windows executable using Pyinstaller from a Linux Machine.
So to distribute executables for multiple operating systems, you'll require a dedicated build machine for each supported OS
Installing Pyinstaller
Make sure that you have a Python interpreter installed on your Windows System before running the PIP command.PIP is installed along with Python Standard Distribution.
You can install Pyinstaller on Windows 10 using the PIP command.(below)
pip install pyinstaller
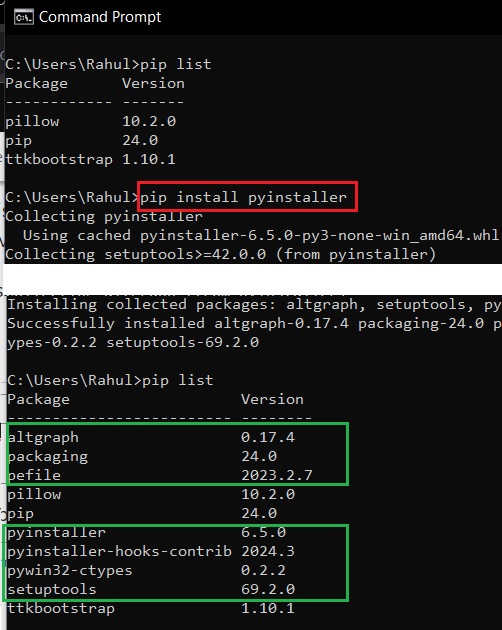
Convert Python (.py) file to Windows (.exe) file
Make sure that all the libraries needed by your Python script are installed on the system in which you are running the Pyinstaller program.
Here we will convert a simple Python script to a Windows executable using the Pyinstaller program.
We will use the below Python script that adds two numbers for our conversion.The code will take two numbers and add them.
# Python script to add two numbers and display the result in command line
#save as add.py
print('\nCalculates Sum of two numbers \n')
num1 = input('Enter Number1 -> ')
num2 = input('Enter Number2 -> ')
sum = float(num1)+float(num2)
print(f'Sum = {sum}')
input('\n\nPress Enter to Exit')
We will save the code as add.py and put inside the folder "test".
Now you can use the pyinstaller to convert the python file to exe by
pyinstaller add.py
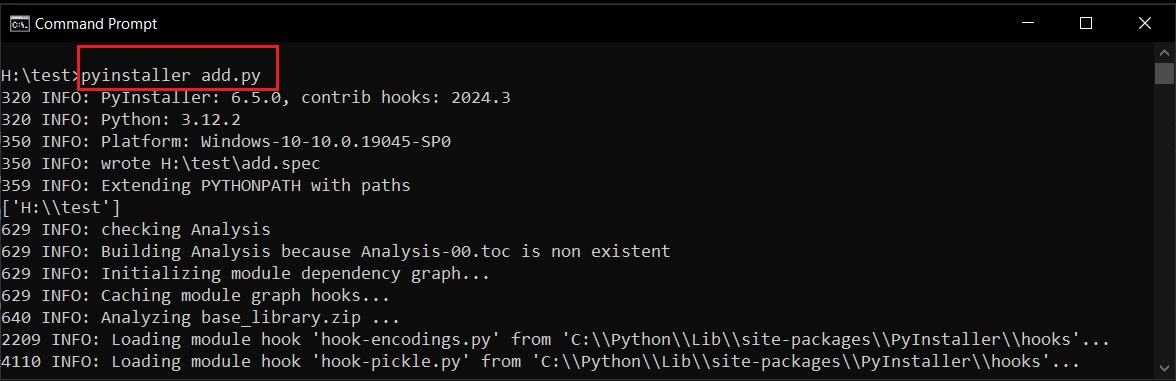
Once the program has finished running we will get the following folder structure inside the test directory.
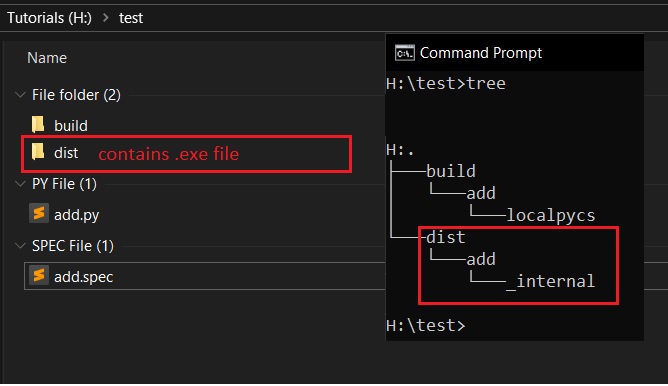
The "build" folder is where PyInstaller stores much of the metadata and internal files necessary for creating your executable. While the contents of the build folder can be helpful for troubleshooting, it's typically not essential to interact with it directly unless you encounter specific issues during the build process. In most cases, you can safely disregard this folder
You can find the executable inside the
test\dist\add
folder.
Here add.exe is the windows executable and _internal contains all the required files (mostly dll files) needed for the add.exe to run.
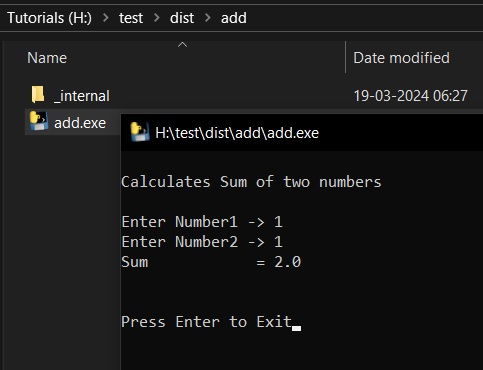
Please note that the add.exe and _internal should be in the same directory for the executable to run.
Creating a Single Windows Executable from Python Script
You can combine the _internal folder with the add.exe to create a single executable by using --onefile flag of pyinstaller as shown below.
pyinstaller --onefile add.py
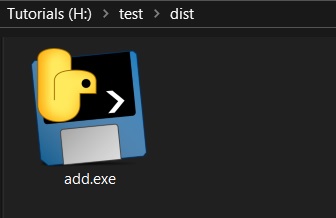
Converting a tkinter python script to .exe file
Here we will convert a tkinter Python GUI script to a single Windows executable using Pyinstaller.
Python tkinter script we will be using is shown below.
import tkinter as tk
from tkinter import font, filedialog
def open_file():
file_path = filedialog.askopenfilename()
if file_path:
with open(file_path, 'r') as file:
text.delete(1.0, tk.END)
text.insert(tk.END, file.read())
def save_file():
file_path = filedialog.asksaveasfilename(defaultextension=".txt")
if file_path:
with open(file_path, 'w') as file:
file.write(text.get(1.0, tk.END))
def change_font():
font_family = font_var.get()
font_size = size_var.get()
text.config(font=(font_family, font_size))
# Create the main window
root = tk.Tk()
root.title("Text Editor")
# Create a Text widget
text = tk.Text(root, wrap="word")
text.pack(expand=True, fill="both")
# Create a menu
menubar = tk.Menu(root)
file_menu = tk.Menu(menubar, tearoff=0)
file_menu.add_command(label="Open", command=open_file)
file_menu.add_command(label="Save", command=save_file)
menubar.add_cascade(label="File", menu=file_menu)
root.config(menu=menubar)
# Font options
font_options_frame = tk.Frame(root)
font_options_frame.pack()
font_label = tk.Label(font_options_frame, text="Font:")
font_label.grid(row=0, column=0)
font_var = tk.StringVar(value="Arial")
font_entry = tk.Entry(font_options_frame, textvariable=font_var)
font_entry.grid(row=0, column=1)
size_label = tk.Label(font_options_frame, text="Size:")
size_label.grid(row=0, column=2)
size_var = tk.IntVar(value=12)
size_entry = tk.Entry(font_options_frame, textvariable=size_var)
size_entry.grid(row=0, column=3)
change_font_button = tk.Button(font_options_frame, text="Change Font", command=change_font)
change_font_button.grid(row=0, column=4)
# Start the GUI
root.mainloop()
Save the file as text_ed.py.
You can create a single file Windows executable by issuing the following command.
pyinstaller --onefile text_ed.py
You can find the exe inside the "dist" folder.
If you click on the single file executable you can see a command window along with the GUI program.
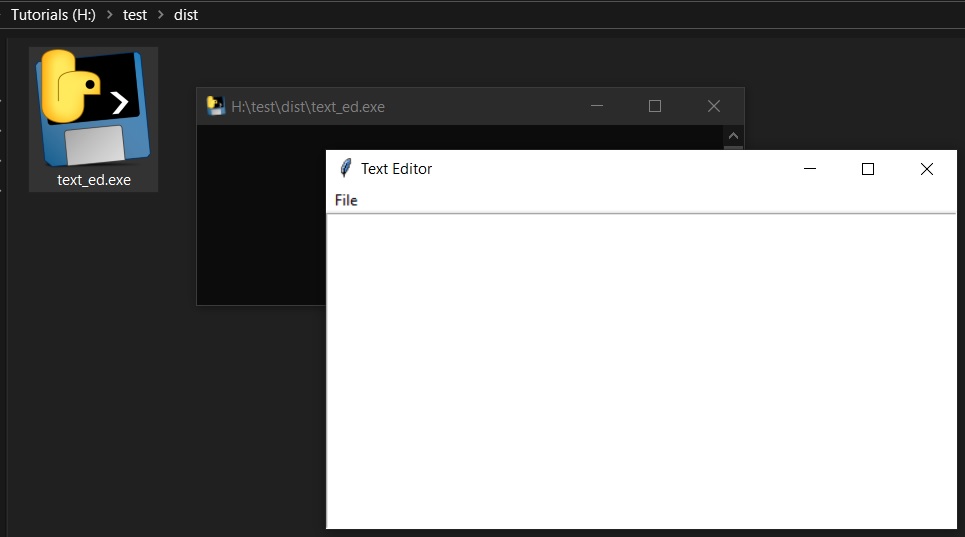
To remove the command window in your tkinter application ,please add --noconsole flag while creating the executable using pyinstaller as shown below.
pyinstaller --noconsole --onefile text_ed.py
Convert ttkbootstrap Python script to a Windows exe
Here we will convert a simple ttkbootstrap python script to windows executable. The code for the ttkbootstrap is shown below.
Make sure that ttkbootstrap is installed on your system before running Pyinstaller.
#ttk_bootstrap_Labels.py
import ttkbootstrap as ttkb
root = ttkb.Window(themename = 'litera')
root.geometry('400x200') # widthxheight
My_Label_1 = ttkb.Label(text = "Helvetica 15",bootstyle = 'primary',font = ('Helvetica',15) ).place(x=10,y=10)
My_Label_2 = ttkb.Label(text = "Ariel 15",bootstyle = 'warning',font = ('Ariel',15) ).place(x=10,y=40)
My_Label_3 = ttkb.Label(text = "Times New Roman 15",bootstyle = 'danger' ,font = ('Times New Roman',15)).place(x=10,y=80)
My_Label_4 = ttkb.Label(text = "Comic Sans MS 15",bootstyle = 'success' ,font = ('Comic Sans MS',15) ).place(x=10,y=120)
My_Label_5 = ttkb.Label(text = "Courier New 15",bootstyle = 'dark' ,font = ('Courier New',15) ).place(x=10,y=160)
root.mainloop()
Save Python file as ttk_bootstrap_Labels.py.
Run Pyinstaller using the following command
pyinstaller --noconsole --onefile ttk_bootstrap_Labels.py
You can find the exe file inside the "dist" folder.
All the above were simple programs, Now we will convert a multithreaded python tkinter script that uses pyserial to windows executable.
The python script is from our tutorial on building a serialport based CSV datalogger
Make sure that Pyserial is installed on your system.before running Pyinstaller.
pyinstaller --noconsole --onefile python-ttkbootstrap-data-logger.py
Here is the result
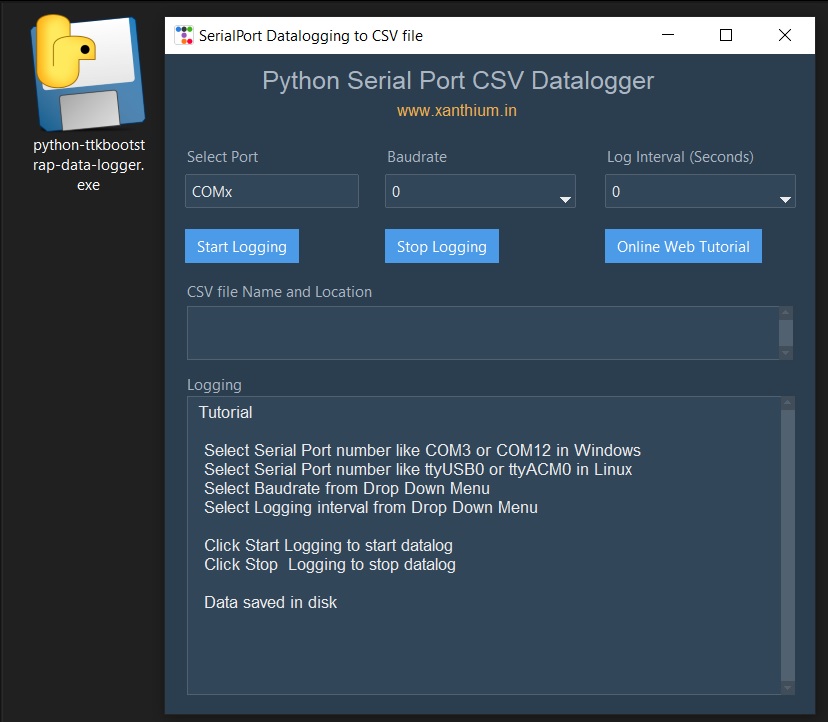
How to add Custom icon to Pyinstaller Generated Windows Executables
In the previous examples, All the icons of pyinstaller generated windows executable looked like the one ,shown below.
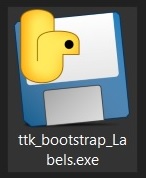
Now lets say we want to add a custom icon to the Pyinstaller generated Windows executable. First we create an icon file using a image editing software like Gimp or Photoshop.Save it with the .ico extension of size 255X255.
Place the icon in the test directory ,same directory as the one containing .py file.
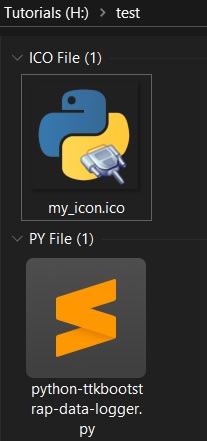
You can then use the --icon flag of pyinstaller to add a custom icon to your python script as shown below.
pyinstaller --noconsole --onefile your_python_script.py --icon=name_custom_icon.ico
no space between the =
--icon=name_custom_icon.ico
In our case
pyinstaller --noconsole --onefile python-ttkbootstrap-data-logger.py --icon=my_icon.ico

Now in the dist folder ,You will find your executable (here python-ttkbootstrap-data-logger.exe ) with the same default pyinstaller icon.
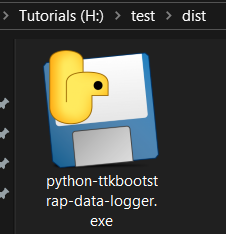
That is because Windows explorer caches the icon files. Please move the executable to a different folder to view the true icon .
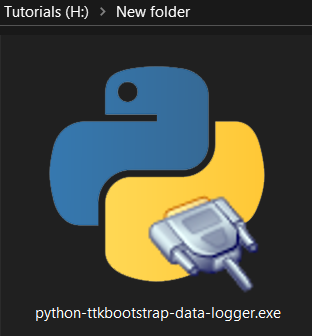
- Log in to post comments